In everyday life, you must have had an experience when a carton of milk stayed in your refrigerator for longer than a while and you forgot to throw it out. What happens next is that you have stale milk in your refrigerator, occupying space, is of no use and can only be harmful if consumed. Similarly, your data in cache is supposed to come with an expiry date too. Any data kept in your cache for long becomes stale (like the milk), is of no use and only occupies space in your cache. And the solution in both the cases is similar: throw it out!
This blog is all about explaining the expiration strategies for keeping the data in your cache fresh and throwing the stale data out.
Cache Data Expiration: The Need and the Concept
Let’s suppose you are running an e-commerce website and you added the information of these products in the cache. For a long period of time, the products’ information is retained in the cache without being changed. But now for some reason, the prices of some products have been updated in the data source. In this case, data inconsistency occurs because your customers are still viewing the older prices which is actually the stale cached data.
NCache provides expiration where you can set a time limit with your data, and once the limit has been reached, the data is no longer valid. Invalidated data needs to be removed from the cache after a pre-defined Clean Interval. It is an interval (set by you) after which all the expired items are automatically removed from the cache. Thus, any item expired is not removed from the cache immediately.
Data Expiration in NCache NCache Details
Types of Expiration in NCache
NCache provides the following strategies to cater to the user’s requirement:
- Absolute/Default Absolute Expiration
- Sliding/Default Sliding Expiration
We will look closely at both of these types and their usages.
Absolute Expiration
In this strategy, an absolute time is specified with the item that needs to be invalidated. The time specified is maintained on the UTC time format, hence, time specified in any zone is converted to UTC time format on the cache server. The time can range from seconds to hours to days to months, and after the time has elapsed, the item is invalidated or expired. Look at Figure 1 for clarity:
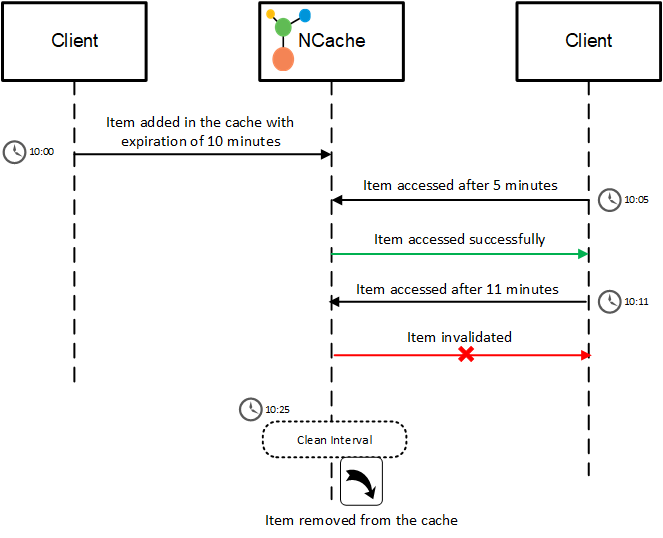
Figure 1: Absolute Expiration in NCache
Use Case: For cases when you can estimate how long any item is required to be retained in the cache. For example, if a limited time product is launched and can not be sold after 24 hours, the item is added with an absolute expiration of 24 hours and after that, the product expires from the cache.
Let us look at the code example that shows adds an item in the cache that expires after 5 minutes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Pre-condition: Cache is already connected // Get product from database against given product ID Product product = FetchProductFromDB(1001); // Generate a unique cache key for this product string key = $"Product:{product.ProductID}"; // Create a new CacheItem for this product with expiry var cacheItem = new CacheItem(product); var expiration = new Expiration(ExpirationType.Absolute, TimeSpan.FromMinutes(5)); cacheItem.Expiration = expiration; cache.Insert(key, cacheItem); |
Sliding Expiration
As the name explains, this strategy keeps the data in the cache as long as it is being used. So, the data that has not been used for a specific time is invalidated. Every time any data added with sliding expiration is accessed, the duration for that data to exist in the cache is extended. For example, if any item with 30 seconds sliding interval, is not accessed for 30 seconds, it is expired. Similarly, if it is accessed within 30 seconds, the life of the item is exceeded by another 30 seconds in the cache.
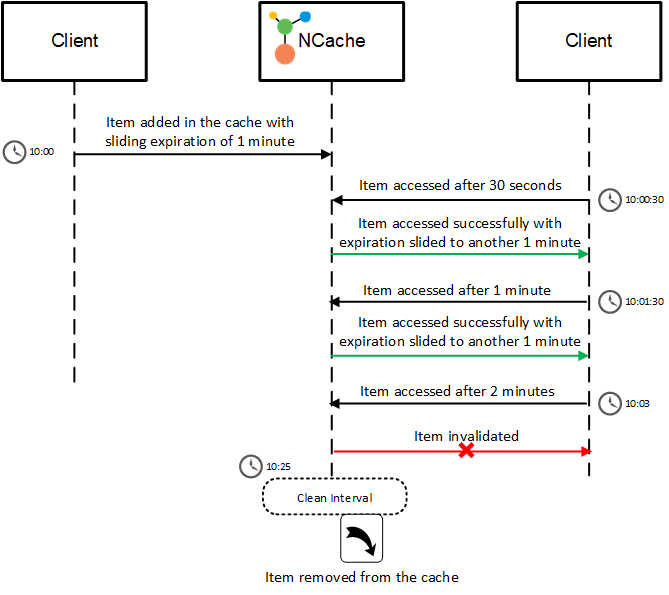
Figure 2: Sliding Expiration in NCache
Use Case: Let’s suppose you have an application that takes the user’s credentials to be accessed. You want to provide access to the user as long as the user is active and using the application. Sliding expiration helps you in such cases where the session can be maintained by keeping track of the active users.
In the example given below, a sliding expiration of 5 minutes is added with the product:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// Get product from database against given product ID Product product = FetchProductFromDB(1001); // Generate a unique cache key for this product string key = $"Product:{product.ProductID}"; // Create a new CacheItem for this product with expiry var cacheItem = new CacheItem(product); var expiration = new Expiration(ExpirationType.Sliding, TimeSpan.FromMinutes(5)); cacheItem.Expiration = expiration; cache.Insert(key, cacheItem); |
Eviction in NCache Absolute Expiration Sliding Expiration
Default Expiration Strategies
NCache also lets you add default expiration to help you save the effort of manually configuring it. These can only be configured using NCache Web Manager or configuration files (shown later in the blog). Following are the default values:
- Default Absolute
- Default Sliding
- Default Absolute Longer
- Default Sliding Longer
The default values by default are 5 seconds for all the expiration strategies. Please refer to Default Expiration explained thoroughly in NCache documentation.
Configure Expiration using NCache Web Manager
You can enable expiration and set the duration using NCache Web Manager. Let me show you how:
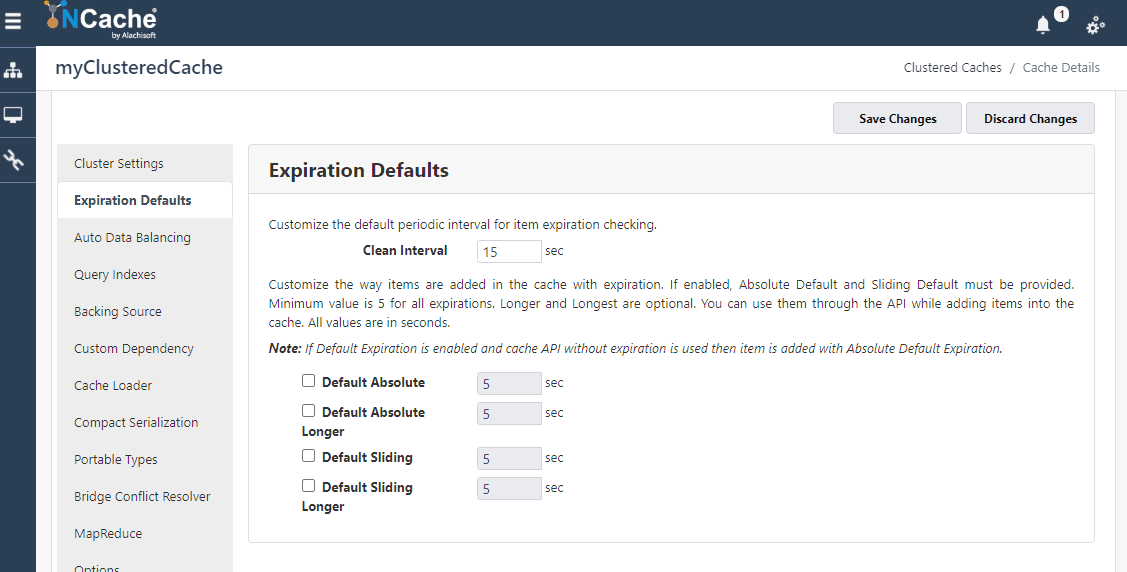
Figure 3: Configure Expiration using NCache Web Manager
Configure Expiration using Configuration files
You can also enable expiration in NCache using the configuration file (config.ncconf) that is installed in NCache install directory by default. Under the <cache-settings> tag, there is an <expiration-policy> tag as shown below:
1 2 3 4 5 |
<expiration-policy enabled="True"> <absolute-expiration longer-enabled="False" longer-value="5" default-enabled="False" default-value="30"/> <sliding-expiration longer-enabled="False" longer-value="5" default-enabled="False" default-value="20"/> </expiration-policy> |
Similarly, you can specify the cleanup interval after which the expired items are removed from the cache as shown below:
1 2 3 |
<cache-settings ... > <cleanup interval="15sec"/> </cache-settings> |
Bulk Removal of Expired Items
All the expired items in the cache are removed in bulk to avoid the performance setback caused by removing individual items. However, you can configure the bulk size of the items to be removed as well as the delay between two consecutive bulk removals of expired data. NCache comes with an Alachisoft.NCache.Service.exe.config file placed in the install directory of NCache and has the following configurable tags:
1 2 |
<add key="NCacheServer.ExpirationBulkRemoveSize" value="10"/> <add key="NCacheServer.ExpirationBulkRemoveDelay" value="0"/> |
Configuring Data Expiration Expiration & Eviction
Facts to Know
- If any item is added in the cache with no expiration (absolute or sliding), it remains in the cache forever until manually removed.
- You will have to restart the NCache service after configuring anything using the configuration files for the change to take effect.
- In NCache, expiration works differently with respect to different topologies, everything is explained thoroughly in Expiration in Clustered Environment.
Summing it Up!
So, everything we have discussed so far sums up to the fact that stale data in your cache requires to be managed efficiently and expiration is the way to do it. It is a technique which expires data from the cache based on the technique provided by you. NCache helps you deal with these things without any hassle.