The advent of distributed caching systems has fixed the problem of databases becoming over-loaded and laggy. Today these distributed caches can handle the load of millions of data transactions taking place per second, but there is a problem.
Most enterprise-level applications rely on changes taking place in some specific portions of this data. These applications have to make critical business decisions based upon these changes and since the size of this data is in thousands of Gigabytes, monitoring specific changes becomes a huge task.
To cater to this problem NCache, an in-memory distributed cache brings with it a feature known as Continuous Query. This blog will explain how Continuous Query works, how you can configure it in NCache, and what benefits you will reap from doing so.
NCache Details NCache Docs Download NCache
Continuous Query to Monitor Changes in NCache
The Continuous Query feature of NCache allows you to keep a track of changes taking place in a selective dataset within the distributed cache cluster. This selective dataset within the cache is defined through SQL-like OQL (Object Query Language) queries. The changes that take place within this dataset are propagated to the applications(that have registered callbacks for events) in the form of Cache Level Events.
Regardless of the fact how big your cache cluster is, you will only be notified of the changes that take place in the dataset which you defined and then registered within the cache. This is also how Continuous Query achieves application decoupling as data is filtered out through OQL queries. This decoupling ensures applications don’t overlap with each other.
It should also be noted that Continuous Query itself doesn’t change your application data. Continuous Query instead provides a mechanism for data to be monitored and shared among applications at runtime through events. The developer then ensures what applications do with this data by registering callbacks for Continuous Query events and defining their business logic.
Continuous Query SQL in NCache Cache Event Notifications
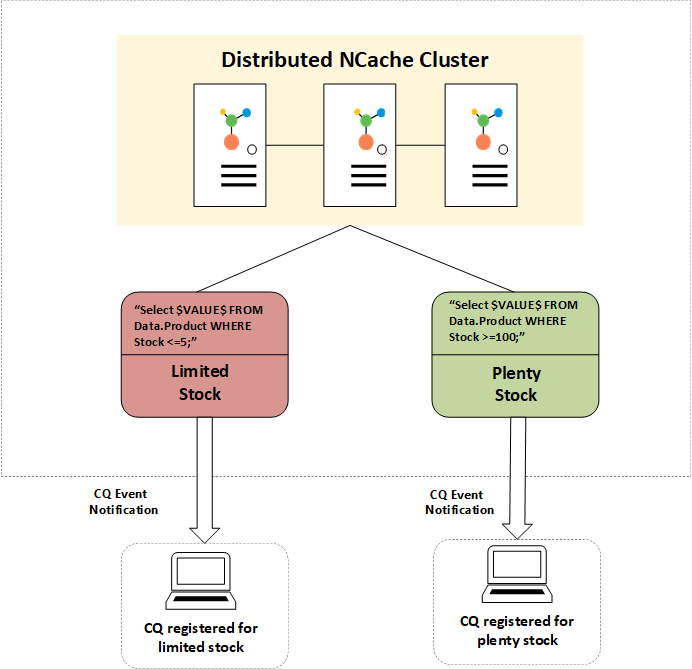
Figure 1: A diagram depicting the working of Continuous Query
Configuring Continuous Query in NCache
It’s easy to configure Continuous Query in NCache. You just have to follow a two-step process. Once you follow these steps, your application will be able to receive notifications against the dataset defined by you and act accordingly.
These two steps are as follows:
Step 1: Register Callback for Events
You need to register a callback for cache level events: ItemAdded, ItemUpdated, or ItemRemoved. Through these events, you will define your business logic that will dictate what your application should do if one of these events is fired.
In the following code example, a callback is registered for the event ItemAdded.
1 2 3 4 5 6 7 8 9 |
static void QueryItemCallBack(string key, CQEventArg arg) { switch (arg.EventType) { case EventType.ItemAdded: Console.WriteLine(“Item has been added”); break; } } |
Step 2: Register Query and Notifications
Once you have registered the callbacks for the events, you have to create a Continuous Query that will specify the criteria for the resultant dataset. The events will fire based on this Continuous Query. After this, the callbacks are registered with the query. Once this is done, the query is registered against the cache server using the RegisterCQ method.
The code example below depicts how all of this happens.
1 2 3 4 5 6 7 8 9 10 11 12 |
string query = "SELECT $VALUE$ FROM FQN.Product WHERE Category = ?"; var queryCommand = new QueryCommand(query); queryCommand.Parameters.Add("Category", "Beverages"); // Create Continuous Query var cQuery = new ContinuousQuery(queryCommand); // Item add notification cQuery.RegisterNotification(new QueryDataNotificationCallback(QueryItemCallBack), EventType.ItemAdded, EventDataFilter.None); cache.MessagingService.RegisterCQ(cQuery); |
Unregister Continuous Query
NCache gives you the option to unregister notifications from the Continuous Query when you no longer require them. This is done using the UnRegisterNotification method.
In the following code example, an event notification is unregistered from a Continuous Query.
1 |
cQuery.UnRegisterNotification(new QueryDataNotificationCallback(QueryItemCallBack), EventType.ItemAdded); |
NCache also gives you the option to unregister the Continuous Query itself from the cache cluster. It is unnecessary to keep it running within the cache when you no longer need it as it consumes resources. To unregister a Continuous Query from the cache, NCache gives you the UnRegisterCQ method.
In the following code example, a Continuous Query is unregistered from the cache server.
1 |
cache.MessagingService.UnRegisterCQ(cQuery); |
NCache: The Best Solution Out There
When it comes to large and complex business applications, data refining is a huge challenge. Stream Processing in NCache caters to this challenge by converting large and complex data into data streams for easy processing.
A popular application of Stream Processing is the Publisher Subscriber model of NCache. However, this comes with the following limitations:
- Messages are not persisted by the applications once they are delivered to the subscribers.
- Data filtration takes place at the client end, making the application architecture more complex.
Continuous Query addresses both of these problems in the following ways:
- By persisting data inside the cache even after processing.
- By filtering data through fairly simple OQL statements at the server end instead of the client end. This ensures a simple application architecture.
Continuous Query Stream Processing Pub/Sub Messaging
Conclusion
NCache is an extremely fast, easy-to-scale, distributed cache that deals with database bottlenecks efficiently and effectively. Just like Continuous Query, Stream Processing, and Pub/Sub Messaging, NCache has many other rich and powerful features which you should try out. These features give you both quantitative and qualitative results. Try NCache now!