Most flourishing e-commerce businesses of today have suffered from a slow website reaction time at some point in the past. This is partly due to a paradigm shift in user preferences – more inclined towards online buying. Therefore, traditional mechanisms have failed to sustain the influx of customer requests.
With the advent of in-memory, linearly scalable, distributed caching solutions like NCache, there have been significant improvements in business response times. Most prospering enterprises have a caching layer part of their system architecture – which has given a substantial boom to businesses initially.
NCache Details NCache Loader Docs NCache Loader Admin Guide
What is a Cache Loader?
Before I move on, let’s consider a scenario – your business plans to introduce a flash sale discount at midnight. That’s when the influx of user requests will begin. So, how about you load your cache with the relevant discount details before the sale officially begins. This way, once you get bombarded with user requests, you’ll be equipped with the relevant information to deal with them.
Enterprise caching solutions like NCache help overcome this initial performance lag by incorporating a special caching feature – cache startup loader to pre-load your cache. NCache exposes an ICacheLoader interface that you can implement and then deploy on the server. Based on your logic, the data is then pre-loaded in the cache as a background process, on start-up.
The NCache ICacheLoader interface exposes the following three methods – Init, Dispose, and LoadDatasetOnStartup which you can custom code based on your business requirements:
1 2 3 |
public void Init(IDictionary<string, string> parameters, string cacheName); public object LoadDatasetOnStartup(string dataset); public void Dispose(); |
Cache Loader Features
Coming with a rich feature set, the NCache start-up Loader helps promote an enhanced user experience. It is developed, keeping the client-facing issues under consideration, while looking for ways to solve them.
To help you form a deeper understanding of the topic, let’s look into the core NCache loader features below:
Easily configurable
The ICacheLoader
interface is easy to configure. You just have to implement it and then deploy your logic via the NCache Manager on the server-side. By enabling the cache loader feature, your code is automatically executed on cache start-up.
Logical data sets
If you are working on a multi-node cache cluster, you can divide your data into logical sets and NCache speeds up the process of data loading by assigning these groups to cluster nodes in a round-robin fashion.
Say, you have a two-node cluster and your business requires you to load two data sets: products
and suppliers
. Simply include them in your interface logic and specify these data sets in your cache configuration. NCache will begin data loading on both of the cluster nodes in parallel.
Below is a sample implementation of the method LoadDatasetOnStartup:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
public object LoadDatasetOnStartup(string dataset) { // Create a list of datasets to load at cache startup IList<object> datasetToLoad; switch (dataSet.ToLower()) { // If dataset is "products", fetch products from data source to load in cache case "products": datasetToLoad = FetchProductsFromDataSource(); // Insert fetched product in the cache foreach (var product in datasetToLoad) { string key = $"ProductID:{product.Id}"; cache.Insert(key, product); } break; // If dataset is "suppliers", fetch suppliers from data source to load in cache case "suppliers": // load suppliers from database and add the cache. break; default: // Invalid dataset } // User context is the time at which datasets were loaded in the cache object userContext = DateTime.Now; return userContext; } |
A dedicated loader service
NCache uses a dedicated loader service on each of the server nodes to help improve the overall cache speed. This is a critical NCache feature because if a large data set needs pre-loading in the cache, the separate loader service ensures that this does not hinder the regular cache performance.
NCache Details ICacheLoader Interface Docs NCache Deploy-Providers Guide
Use NCache Refresher to Re-load Cache Data
While we know how helpful a preloaded cache can be, but there is a significant chance of the cache data going stale if there are periodic updates at the backend data source. In such scenarios, the data populated in the cache does not stay meaningful – failing to fulfill the purpose of a pre-loaded cache altogether.
Continuing with the e-commerce example from the previous section, say that in the first phase, you were providing a flat 25% off on selected items, but suddenly, you decide to increase the discount percentage to 50% and apply it to the entire stock – what happens to the existing cache data? It’s now outdated – which means you need a mechanism to refresh the relevant data set in the cache.
NCache has a solution for such situations – It allows its users to refresh the cache periodically with the latest data from the source. You can do this by either scheduling your data sets or by invoking the refresher on demand.
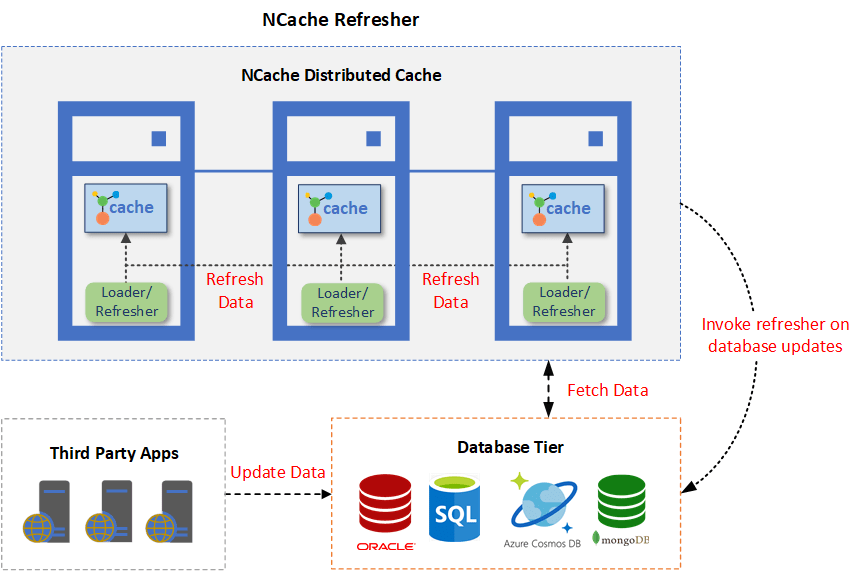
Figure 1: NCache Refresher
If you can predict when your cache will need to be refreshed, you can implement the RefreshDataset
method of the interface and choose an appropriate time interval which can be hours, days, weeks, or months.
Below is a sample implementation of the method RefreshDataset:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
public object RefreshDataset(string dataset, object userContext) { DateTime? lastRefreshTime; switch (dataset.ToLower()) { // If dataset is "products", fetch updated products from data source case "products": lastRefreshTime = userContext as DateTime?; IList<Product> productsToRefresh = FetchUpdatedProducts(lastRefreshTime) as IList<Product>; // Insert updated products in the cache foreach (var product in productsToRefresh) { string key = $"ProductID:{product.Id}"; CacheItem cacheItem = new CacheItem(product); _cache.Insert(key, cacheItem); } break; // If dataset is "supplier", fetch updated suppliers from data source case "suppliers": lastRefreshTime = userContext as DateTime?; // fetch all suppliers updated since ‘lastRefreshTime’ and insert in cache. break; default: // Invalid dataset } // User context is the time at which datasets were refreshed userContext = DateTime.Now; return userContext; } |
If, on the other hand, you are not sure when your cache data will be outdated, you can implement the GetDatasetsToRefresh method in the ICacheLoader
interface to programmatically find the datasets that have changed and refresh them. You can also use the PowerShell Invoke-RefresherDataset cmdlet to refresh the cache on demand.
1 |
public IDictionary<string, RefreshPreference> GetDatasetsToRefresh(IDictionary<string, object> userContexts); |
Conclusion
A Cache loader is not just an option anymore – it’s a must-have feature for enterprises looking to expand their clientele globally because in this fast-paced, competitive era, the front face of your business can never go down. So, if you are looking for a distributed caching solution like NCache to help grow your business, get in touch with us and let our technical experts help you out!