Looking around us, we see hundreds and thousands of real-time web applications that thrive on availability, reliability, and constant feedback. For example, with the Premier League (a top level of the English football league system) matches currently going on, many people have shown interest in betting against these matches.
As a result, the leading real-time web apps using .NET Core SignalR for sports betting have a high demand and an increased transaction load. These apps certainly cannot afford to slow down during these peak usage times, anhttps://www.alachisoft.com/resources/docs/ncache/prog-guide/aspnet-signalr.htmld they need to keep performing better. In other words, these real-time web apps need scalability.
If you have a .NET Core real-time web app that manages functionalities like sports betting, stock market, or foreign exchange market and is currently in demand – requiring high-performance numbers- then you need scalability, as well. Increasing the number of servers to divide the client load could benefit you in multiple ways. And what better way to do that than by using NCache, a native .NET, distributed in-memory caching solution?
NCache Details NCache SignalR Docs NCache SignalR Backplane
Using NCache as Backplane to Achieve Scalability
Fortunately for you, NCache has implemented SignalR backplane for high-traffic, ASP.NET Core real-time web applications running in load-balanced, multi-server web farms. A backplane is a shared repository source for your application. This resource has proven itself to be a reliable solution to all your request-response problems. NCache, as a SignalR backplane, acts as a communal message bus for all the web servers that are a part of your .NET Core application.
Using NCache as a SignalR backplane can provide the following functionalities to you:
- Persistent connection: This connection means there is no need to create unnecessary connection calls every time.
- Invoke two-way methods: Not only can clients invoke server methods, but NCache SignalR also allows servers to invoke client methods. This functionality omits the need to refresh your session every time.
- Built-in transport layer: NCache provides a built-in transport layer – making TCP connections simple and more reliable.
- Real-time updates: It allows real-time updates without any reloading.
- Ensures response delivery: NCache as backplane ensures that the message delivers to every connected client.
Applications that run on the request-response model should use NCache as a backplane to boost their performance.
NCache Details NCache SignalR Docs NCache SignalR Backplane
How NCache as Backplane works: A Quick Example
SignalR applications greatly benefit from NCache backplanes in a multi-server environment. To understand how NCache backplane works, let me take you to a real-time application providing sports betting environment. Let’s say there are multiple web servers delivering score updates to all interested clients. Here, we use NCache as the backplane. Let us see how NCache as a backplane solves scalability problems.
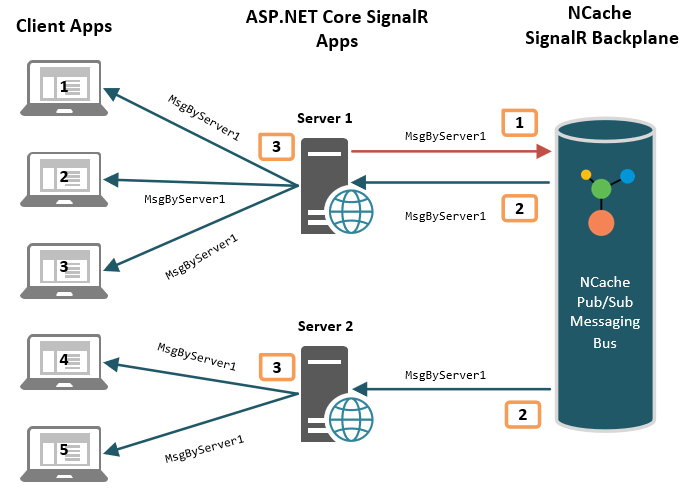
Figure 1: NCache as Backplane for SignalR Apps
- Sending updates: A source, the scoreboard, sends updates over a web server.
- Placing updates on the backplane: That server then sends updates to NCache’s backplane.
- Backplane broadcasts updates: NCache broadcasts the updates to all the servers holding the information.
- Servers delivering updates: The server sends these updates to all connected clients.
In this way, the NCache backplane ensures that all the clients receive the updates. This includes all those using this sports betting real-time app – regardless of their connection server. And during this process, if you feel like the transaction load is increasing on one or multiple servers, NCache allows you to add servers at runtime without stopping the cache. So, using NCache as a backplane for your .NET Core real-time web application provides scalability and high performance.
NCache Details NCache SignalR Docs NCache SignalR Feature
NCache Configuration
Everything you need to know to configure NCache as a backplane in your application is mentioned below.
Your first step should be modifying your .NET Core application’s appsettings.json file with the following credentials to update NCacheConfiguration accordingly.
1 2 3 4 |
"NCacheConfiguration": { "CacheName": "DemoCache", "ApplicationID": "scoreboardApplication" } |
After updating your application’s appsettings.json, your second step should be adding the following line of code in Startup.cs to start using NCache as a backplane in your ASP.NET Core application.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
public class Startup { public void ConfigureServices(IServiceCollection services) { // ... services.Configure<NCacheConfiguration>(Configuration.GetSection("NCacheConfiguration")); services.AddSignalR().AddNCache(ncacheOptions => { ncacheOptions.CacheName = Configuration["NCacheConfiguration:CacheName"]; ncacheOptions.ApplicationID = Configuration["NCacheConfiguration:ApplicationID"]; }); } } |
For more information on NCache backplane implementation in your application, please refer to our documentation at Using NCache Extension for SignalR Core.
NCache Details NCache SignalR Docs NCache SignalR Feature
NCache Backplane Features
A good business always indulges every possible way of providing its customers with the best solution imaginable. NCache offers multiple methods to achieve extreme scalability.
The following are some of the distinct features provided by NCache as backplane.
Pub/Sub for Durability
Pub/Sub messaging is an NCache feature that allows fast and durable exchange of messages among multiple applications. NCache backplane uses Pub/Sub messaging as a special constituent to send and receive messages in a web farm. Using a strong feature like Pub/Sub in NCache backplane increases the application’s performance numbers exponentially.
High Availability through NCache Backplane
NCache is a self-healing distributed clustered architecture, so, even if a node goes down, there is always another node present to intelligently replicate orphaned data. This prevents any data loss from occurring making your .NET Core application highly available and extremely reliable.
Extremely Fast and Scalable Real-Time Apps
NCache is an in-memory solution, meaning that the cache resides inside your system making your application fast and durable. On top of being fast, NCache is also linearly scalable, allowing you to add servers at runtime. This prevents any bottlenecks in your application even under massive data load.
NCache, a Native .NET Solution
NCache is the only true native .NET dedicated, distributed cache available in the market today. The servers and the clients provided are all native .NET, making the cost of deployment and maintenance a whole lot cheaper. Hence, NCache provides a natural habitat for your .NET Core application.
Did you find the solution that you were looking for? If yes, then do check out more of what NCache has to offer. Have fun NCaching!