ASP.NET has now become a really popular technology for web apps and more people are developing high traffic applications in it. To handle the higher traffic, these ASP.NET apps are deployed in load-balanced web farms where you can add more servers as your traffic load increases.
But a problem occurs; the database and your data storage cannot scale in the same fashion to handle the higher traffic loads. So, what you get is a bottleneck where your ASP.NET application slows down and can even grind to a halt. In such situations, data caching is an excellent way of resolving this database and data storage bottleneck. Caching allows you to store application data close by and reduce those expensive database trips.
NCache Details NCache Docs ASP.NET Caching
What is ASP.NET Cache?
ASP.NET Cache allows you to cache application data and is a fairly feature-rich cache including the following features:
- Expirations: Automatic absolute and sliding expirations.
- CacheDependency: To manage data relationships in the cache.
- SqlCacheDependency: To synchronize cache with the database.
- Callbacks: To be notified when items are updated in the cache.
Here is some sample code showing ASP.NET Cache usage
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<span class="kwrd">using</span> System.Web.Caching; <span class="rem">// Create a key to lookup in the cache</span> <span class="rem">// The key for will be like “Employees:PK:1000”</span> <span class="kwrd">string</span> key = <span class="str">"Employee:EmployeeId:"</span> + emp.EmployeeId.ToString(); Employee employee = (Employee)Cache[key]; <span class="kwrd">if</span> (employee == <span class="kwrd">null</span>) { <span class="rem"> // item not found in the cache. load from db</span> LoadEmployeeFromDb(employee); <span class="rem"> // Now, add it to the cache for future reference</span> Cache.Insert(key, employee, <span class="kwrd">null</span>, Cache.NoAbsoluteExpiration, Cache.NoSlidingExpiration, CacheItemPriority.Default, <span class="kwrd">null</span> ); } |
Cache Dependency SQL Dependency Data Expiration
ASP.NET Cache Limitations in Web Farms
Despite very useful caching features, ASP.NET Cache has some serious limitations. Some of them are given below:
- Does not synchronize across a server or worker processes: NET Cache does not synchronize across multiple servers or even multiple worker processes. So, you cannot use it in a web farm or even a web garden unless your data is read-only whereas you need to cache all kinds of data, including one that changes somewhat frequently.
- Cache size limitation: You cannot grow the ASP.NET Cache to be more than what one ASP.NET worker process can contain. For 32-bit systems, this is 1GB and that includes app code as well. Even for 64-bit systems, the size cannot scale.
Use ASP.NET Cache Compatible Distributed Cache
The way to work around these limitations of ASP.NET Cache is to use a distributed cache like NCache for web farms. NCache provides the same features that ASP.NET Cache plus more. But, as a distributed cache, NCache easily synchronizes across multiple servers. Here are some benefits you get from NCache:
- Scale’s transaction load very nicely: You can keep adding more cache servers to the cache cluster as your web farm grows from 2 to 200 servers. NCache never becomes a bottleneck in handling more traffic.
- Scale’s data storage nicely: As you add more cache servers, your cache storage capacity grows due to Partition Cache topology.
- Replicates data for reliability: You can ensure that no data loss occurs even if a server goes down because data is replicated to other servers.
- Dynamic self-healing cache cluster: NCache provides 100% uptime through this. And, you can add or remove cache servers at runtime without stopping the cache or your application.
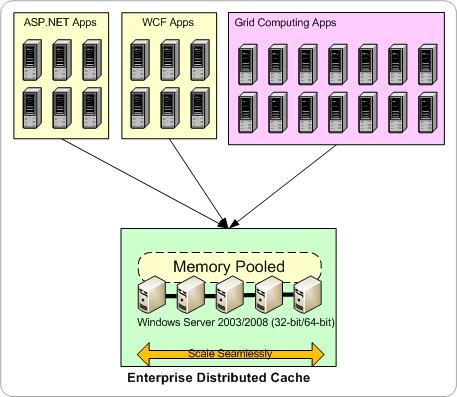
Figure 1: NCache as ASP.NET Cache
Conclusion
Well, if you have an ASP.NET application running on a web farm, take a look at NCache and see how it will help improve your application’s performance and scalability.
You can always drop us an email at sales@alachisoft.com
Appreciate it for the excellent writeup. Anyway, how could we communicate?
I’ve used NCache during one of my projects and results were very impressive. highly recommended !!!