Nowadays, most of the user applications that rely on heavy database calls are incorporating distributed caching for better performance and linear scalability. For this purpose, the user would want that whenever changes occur in the database, the change is immediately reflected in the cache as well. NCache being an in-memory distributed cache, provides us different techniques for similar purposes to keep data fresh in cache.
Firstly, NCache provides Expiration where data is automatically removed from cache after a pre-defined interval. Secondly, comes the strategy of Cache Dependency on Database where the data in cache remains sync with the database and is invalidated whenever it gets changed. Likewise, comes another mechanism that is Cache Refresher which runs in the background on scheduled intervals and keeps the data fresh and updated in a controlled manner.
This blog is all about explaining the different strategies of Cache Refresher and how it keeps the data fresh in cache.
NCache Details Expiration Docs Cache Loader and Refresher
Cache Refresher: Overview and Concept
Let’s suppose user runs a video streaming channel and cached some new information along with their respective videos in the cache. For a longer period of time, the videos in the cache remain unchanged but now for some reason, certain new videos get added and others get updated in the database. This causes the users to view the older videos (stale data) which already has been updated.
NCache provides Cache Refresher feature which keeps data in the cache fresh by refreshing it at a specific refresh interval. Refreshing of cache data includes adding, updating, and removing it whenever data changes in the data source.
To configure Cache Refresher, the user first needs to implement Cache Startup Loader. This loader is used to pre-load the data in the cache on cache startup in the form of datasets which represent a way to group different types of data together to achieve parallelism. Every time the cache starts, Cache Loader automatically fetches data from the data source against the configured datasets. To keep this pre-loaded data fresh and in sync with the updated data in data source, Cache Refresher is used which refreshes these datasets separately after a scheduled time interval.
Different Properties of Cache Refresher
There are some properties for Cache Refresher that user needs to know before starting with the implementation. Explained below are their details needed to be kept in mind.
- Datasets: A dataset is a way for user to group different types of data so that they can load or refresh them separately at different intervals or events to achieve parallelism.
- Dataset Scheduling: Datasets are refreshed according to a dataset schedule. This schedule can be: Daily Time, Daily Interval, Weekly and Monthly. All of these, which, in their unique implementation, decide the exact time after which a dataset needs to be refreshed. Each dataset can have its own refresh schedule. An example can be that, refresh video regarding dataset bakery products on every Sunday at noon for each month. For further details, refer to Dataset Scheduling in Docs.
- Refresh Interval: It is an interval after which a thread runs to check the datasets that are ready to be refreshed when their scheduled time has arrived. Each dataset has a different scheduled refresh interval but this interval remains the same at cache level. This interval can be set to a minimum of 15 minutes and a maximum of 60 minutes respectively.
Cache Loader and Refresher Docs Cache Dependency Docs
How to Implement Cache Refresher?
For implementation with Cache Refresher, first the user needs to configure the ICacheLoader interface. Secondly, NCache calls the implemented LoadDatasetOnStartup method to load data into the cache. It then uses the RefreshDataset method to refresh the data loaded in the cache by the Cache Loader.
Let’s suppose the user has two videos in the database, one regrading bakery products and the other clothing products. The following implementation pre-loads videos into the cache and then for any update refreshes the data on a separate refresh interval for both.
Initialize Cache and Connection
Init method is called on Cache Startup to configure the connection. Below is a sample implementation of Init method of the ICacheLoader interface. Here we open an SQL connection and initialize a cache with the given name by the user.
1 2 3 4 5 6 7 8 |
public void Init(IDictionary<string, string> parameters, string cacheName) { string connectionString = parameters["connectionString"] as string; connection = new SqlConnection(connectionString); connection.Open(); cache = CacheManager.GetCache(cacheName); } |
Load Datasets in Cache
In the second step, the user uses LoadDatasetOnStartup, a method called on cache startup to load specified datasets into the cache from the database to prefill the cache.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
public object LoadDatasetOnStartup(string dataset) { // Create a list of datasets to load at cache startup IList<object> datasetToLoad; switch (dataSet.ToLower()) { // If dataset is "products", fetch products from data source to load in cache case "products": datasetToLoad = FetchProductsFromDataSource(); // Insert fetched product in the cache foreach (var product in datasetToLoad) { string key = $"ProductID:{product.Id}"; cache.Insert(key, product); } break; default: // Invalid dataset } // User context is the time at which datasets were loaded in the cache object userContext = DateTime.Now; return userContext; } |
Refresh Loaded Datasets
Thirdly, to refresh datasets at periodic intervals, user should provide the implementation of RefreshDataset. Here, the user should either update the already pre-loaded data loaded by Cache Loader, add new data or remove the data from that particular dataset.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
public object RefreshDataset(string dataset, object userContext) { *DateTime? lastRefreshTime; switch (dataset.ToLower()) { // If dataset is "bakery products", fetch updated products from data source case "bakery products": lastRefreshTime = userContext as DateTime?; IList<Product> productsToRefresh = FetchUpdatedProducts(lastRefreshTime) as IList<Product>; // Insert updated products in the cache foreach (var product in productsToRefresh) { string key = $"ProductID:{product.Id}"; CacheItem cacheItem = new CacheItem(product); _cache.Insert(key, cacheItem); } break; default: // Invalid dataset } // User context is the time at which datasets were refreshed userContext = DateTime.Now; return userContext; } |
Implement Poll-Based Refresher
Now, comes the method GetDatasetsToRefresh, which is called at every refresh interval and should only be provided if user wants to use their own custom logic to control the refresh time for different loaded datasets. Those datasets returned are either refreshed immediately or within the same day as told.
Refresh Dataset on Demand
Finally, user also has the option to refresh their pre-configured datasets at runtime through the Invoke-RefreshDataset cmdlet. Here, datasets can be refreshed immediately or within the next 24 hours using the RefreshPreference
option. An example is shown below where dataset product is immediately refreshed on demoClusteredCache
on server 20.200.20.11.
1 |
Invoke-RefresherDataset -CacheName demoClusteredCache -Server 20.200.20.11 -Dataset product -RefreshPreference RefreshNow |
Configure Cache Loader and Refresher through NCache Web Manager
Once the user has implemented Cache Startup Loader and Refresher, he/she can configure both through Web Manager and set the refresh interval according to their requirements. Below is how it can be done.
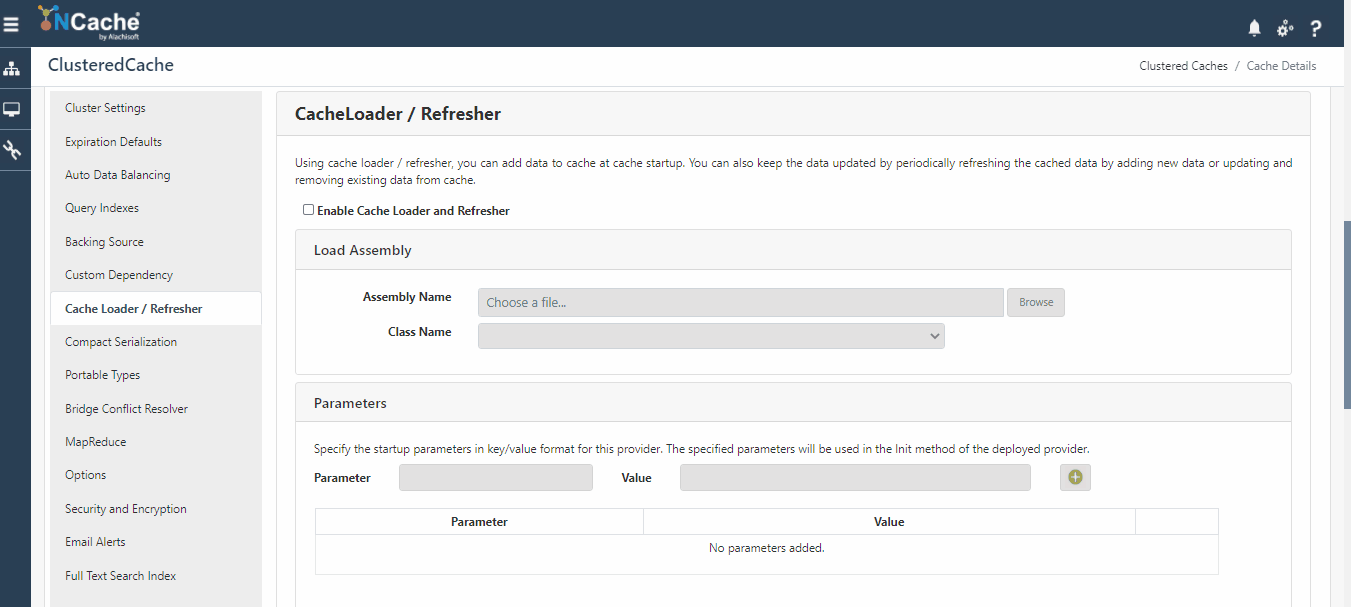
Figure:1 How to configure Cache Refresher and Loader through NCache Web Manager
Configure Cache Loader and Refresher Configuring Data Expiration
Concluding the Blog!
Coming towards the end, everything that has been discussed so far tells that the cache needs to be updated efficiently and Cache Refresher is the best way to do it. It updates the cache data if any change occurs in the database in a very systematic manner. Just like Cache Refresher, NCache provides many interesting features that can be performed without any inconvenience. Check out our website for more details!
NCache Details Download NCache Edition Comparison