NCache as we know by now, stores data in the cache for better performance and achieving high performance and scalability. We have seen NCache doing wonders when it comes to fast access of data for your application’s increased performance, however, we still need to learn what kind of data does NCache store in the cache. Like databases, NCache lets you add data by following simple steps and is very flexible when it comes to data addition and provides a bunch of data types that cater to almost every kind of application’s needs. This blog is all about the forms in which NCache stores your data in the cache-store by providing maximum ease to you.
Key-Value Pairs
For starters, NCache is a key-value store that clearly states that NCache stores data such that there is a key against that value. A key is a string-based identifier and has a following list of properties:
- A key is supposed to be unique and there is no duplication of key allowed by NCache.
- A key cannot be null.
- Key is case sensitive.
A value is the value of the object stored in the cache. We will look at all the supported types in the blog later. This explains the key-value pair where key is used as the identifying attribute for the value stored against it. Figure 1 illustrates how data is added and stored in the cache:
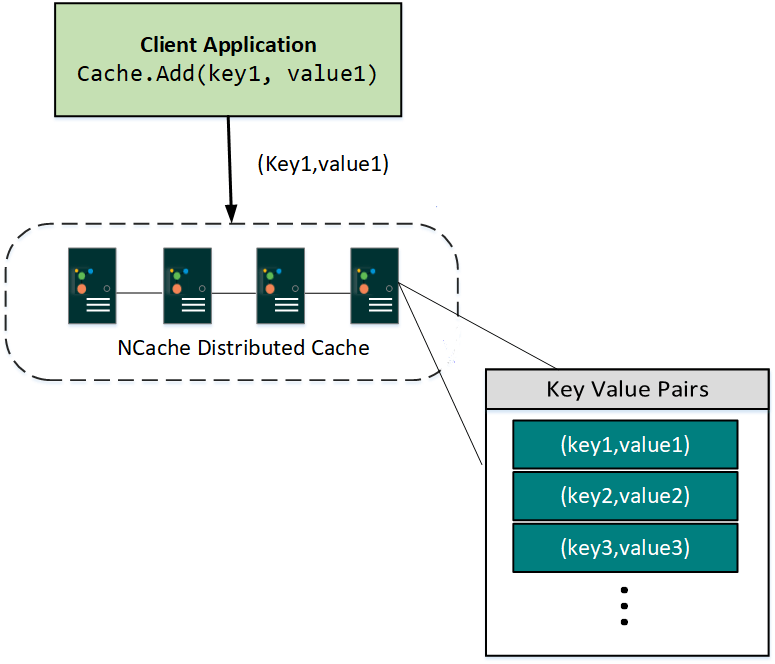
Figure 1: Data in NCache
Supported Data Types in NCache
NCache supports the data in the form of objects. These objects can be in any of the following forms:
- Primitive types
- Custom Class Object
- Data Structures
- JSON Data
Cache Keys and Data Overview Adding Data to Cache NCache Dynamic Clustering
Primitive Data Types
NCache supports all .NET primitive data types. You can add a string as a value against a key. Following are the types supported by NCache:
byte/sbyte | short/ushort | object | string | double | bool | TimeSpan |
int/uint | long/ulong | char | float | decimal | DateTime |
Let us quickly walk through a brief code example showing the addition of a string against the key in the cache:
1 2 3 4 5 6 7 8 |
// Specify the customer name as string string customerName = "John Wick"; // Generate a unique cache key string key = "Customer"; // Add the string to cache cache.Add(key, customerName); |
Custom Class Object
Custom class object refers to a .NET or Java class object e.g. Products or Orders or Customers etc. The only and a very important condition to add a custom class object to your cache is to mark the data serializable. You can serialize the class in the cache by either:
- Adding the .NET Serializable attribute in your custom class or
- Using Dynamic Compact Serialization that is a framework provided by NCache providing cost-effective serialization for registered classes dynamically.
However, unserialized data cannot be added to the cache. Look at the Serialization section for more detail on cache data serialization.
Let us add a custom class object in the cache in the code below. Before adding the data to the cache mark it serialized like shown:
1 2 3 4 5 6 7 8 |
[Serializable] public class Product { public int ProductID { get; set; } public string ProductName { get; set; } public string Category { get; set; } public int Price { get; set; } } |
1 2 3 4 5 6 7 8 |
// Get product from database against given product ID Product product = FetchProductFromDB(1001); // Generate a unique cache key for this product string key = $"Product:{product.ProductID}"; // Add Product object to cache CacheItemVersion version = cache.Add(key, product); |
Data Structures
NCache being distributed in nature offers the functionality of conventional data structures that allows the addition, removal and retrieval of data without affecting the data consistency. Following are the data structures supported by NCache:
- Distributed List: Native .NET implementation of the IList interface. For example, on an e-commerce platform, customers adding/removing items can be done using a distributed list.
1 |
IDistributedList list = cache.DataTypeManager.CreateList(key, dataTypeAttributes); |
- Distributed Queue: The first-in-first-out (FIFO) implementation for items known as Distributed Queue. For example, sentiment analysis done by an intelligence agency to filter out threatening tweets can use a distributed queue to store the tweets in it.
1 |
IDistributedQueue queue = cache.DataTypeManager.CreateQueue(key); |
- Distributed Hashset: An unordered datatype implementation where the values of a set are unique and distinctive. For example, if you have an online book store, HashSets can help you identify which user is interested in which books or has bought how many books.
1 |
IDistributedHashSet userSetMonday = cache.DataTypeManager.CreateHashSet(mondayUsersIds); |
- Distributed Dictionary: A key-value pair that is a native .NET implementation of the IDictionary interface. A value is held against a certain key, for example, you can store login credentials i.e. the user name and the password in the form of a distributed dictionary in a distributed cache environment.
1 |
IDistributedDictionary<string, Product> dictionary = cache.DataTypeManager.CreateDictionary<string, Product>(key); |
- Distributed Counter: A data structure used to increment or decrement the value easily. For example, to keep count of the views a webpage gets per hour or per day can easily be implemented using distributed counter.
1 |
ICounter counter = cache.DataTypeManager.CreateCounter(key, initialValue); |
Look at the code below to get the idea of how to use distributed list in NCache where a list of products is added in the cache:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Specify unique cache key for list string key = "ProductList"; // Create list of Product type in cache IDistributedList list = cache.DataTypeManager .CreateList(key); // Get products to add to list Product[] products = FetchProducts(); foreach (var product in products) { // Add products to list list.Add(product); } |
JSON Data in NCache
JSON is a lightweight readable language used by the applications for being extremely fast and supported by all major JavaScript frameworks. NCache lets you add your JSON data easily in the cache-store by providing a few classes derived from a base class JsonValueBase. Let us look at the classes provided by NCache:
- JsonObject: This class is for unordered name-value pairs where name is the name of the object and value is the value of the JSON object that can be of any primitive type.
- JsonValue: This class represents the primitive data types in JSON’s conventions such as string, integer, or DateTime.
- JsonArray: This class represents a collection of items and represents JArray in NCache’s domain.
- JsonNull: This class represents NULL value in JSON’s standards.
The code below shows the usage of JSON in NCache with code example. The code example shows a JsonObject formed from a string and inserted into the cache.
1 2 3 4 5 6 7 8 9 10 11 12 |
var product = GetProductFromDB(); string jsonString = $@"{{ 'ProductID': { product.ProductID}, 'ProductName': '{product.ProductName}', 'Category': '{product.Category}', 'UnitsAvailable' : { product.UnitsAvailable} }}"; JsonObject jsonObject = new JsonObject(jsonString); cache.Insert(key, jsonObject); // Fetch the jsonObject previously added var jsonObject = cache.Get(key); |
JSON Support in NCache Distributed Data Structures Adding Data to Cache
Location Affinity
An added feature of NCache for providing increased performance is Location Affinity. To achieve higher performance and better results for your application, NCache gives you the control of keeping the data of two different classes on the same node. This creates an affinity between different items belonging to different classes, hence saving the matching cost while fetching those items. All you need to do is add the items having an affinity with similar braces {} hence ensuring that the items exist on the same node. For example, Order_{Product:1001}” shows affinity between the orders belonging to the product object with the product key 1001.
Let me show you a code example where Products and Orders are added on the same node using location affinity.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Product product = FetchProductFromDB(1001); string productKey = "Product:1001"; var productCacheItem = new CacheItem(product); // Add CacheItem to cache CacheItemVersion version = cache.Add(key, productCacheItem); Order order = FetchOrderFromDB(17); // Unique order key for this order using Location Affinity syntax // This will create an affinity for this orderKey with the respective productKey string orderKey = "Order_{Product:1001}"; var orderCacheItem = new CacheItem(order); // Add order with Location Affinity to cache CacheItemVersion version = cache.Add(key, orderCacheItem); |
Conclusion
In conclusion, NCache provides flexible ways to add data into the cache accommodating your application’s requirements. All you need to do is use a simple API in order to add the data in the cache. The best part about NCache is that with every new version, the addition of data becomes easier and more efficient with the support of new features. You can check out other cool features of NCache here.